Comments in JavaScript
As developers, we write source code that focuses on how the program runs. But source code is also something that we often read, and the ability to have non-executable notes as part of our source code is invaluable. We’re able to do this because the JavaScript language offers syntax that allows us to write various kinds of comments as part of our code base.
New developers will especially enjoy this capability, because they are the ones striving to understand the executable portions. The ability to inject comments can help in the process of explaining to themselves how the code works.
Single-Line Comments
Single-line comments are ideal for short descriptions. A double-slash (//
) is used to indicate the start of a comment that continues until the end of the current line.
As a guideline, single-line comments are best placed either at the end of a line or on the line prior to the code being described. Here are some samples.
let step = 0; // A global variable to track our current step
// TODO: Add parameter to suppress step indicatorconst appendLine = function(someText) { // Increment the step value after getting the current step's letter let letter = `${offsetStepA(step++)}) `; const sandbox = document.querySelector('output#sandbox'); sandbox.innerHTML += '<br>'; sandbox.innerHTML += letter + someText;}
const offsetStepA = function(offset) { let step = 'A'; let characterCode = step.charCodeAt(0); characterCode += offset; // same as characterCode = characterCode + offset; let text = String.fromCharCode(characterCode); return text;}
As you can see above, single-line comments are great during the development process for pointing out unfinished tasks (// TODO:
).
Visual Studio Code includes keyboard shortcuts to quickly add or remove single-line comments, as shown in the following animation.
- Comment - Ctrl+k, Ctrl+c
- Un-Comment - Ctrl+k, Ctrl+c
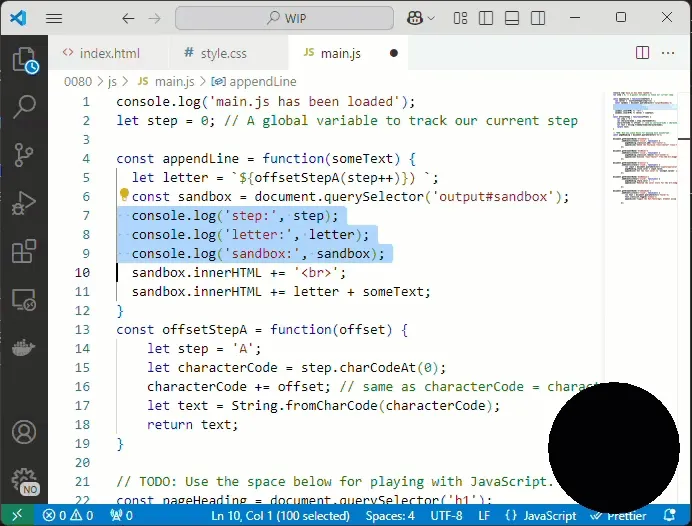
Multi-Line Comments
Multi-line comments are ideal for longer descriptions of blocks of code. The start of a multi-line comment is indicated by a slash followed by an asterisk (/*
). The end of the comment is indicated by the reversal of the opening: an asterisk followed by a slash (*/
).
Multi-line comments are best used at the top of a script file to denote things such as authorship, copyright, etc. They can be used anywhere in your code, but should only be used sparingly.
The following is an example of a multi-line comment.
/* File: gradebookParser.js Author: Stewart Dent Notes: Relies on Papaparse for core CSV parsing. See https://www.papaparse.com/*/
const cellDelimeter = '|';// ... more code ...
JSDoc
JSDoc is a documentation generator built on top of JavaScript’s native multi-line comment syntax. It provides developers with intellisense while writing code. This is possible through the support of modern code editors such as Visual Studio Code.
JSDoc comments begin with a slash and two asterisks (/**
) and end the same way as multi-line comments (*/
).
/** * Displays HTML in the `<code id="output">` element. * @param {string} htmlMarkup A string value that can include HTML markup * @param {boolean} append True if you wish to append the HTML, false if you wish to replace it */const outputLine = function(htmlMarkup, append) { const out = document.getElementById('output'); htmlMarkup += '<br />'; if(!!append) { out.innerHTML += htmlMarkup; } else { out.innerHTML = htmlMarkup; }}
JSDoc is also a command-line tool that can be leveraged to generate HTML documentation of our code. For example, when the command jsdoc something.js
is run on the command line for the above sample, it produces the following HTML file in a folder named out/
.
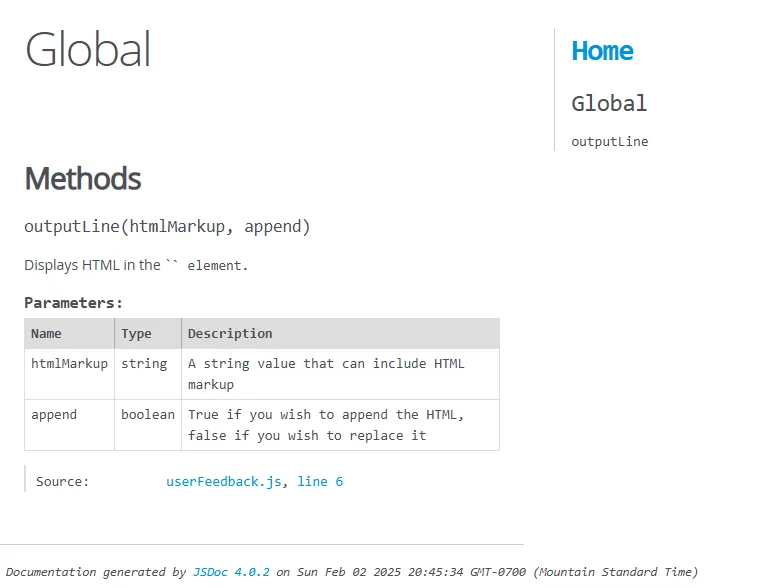
For more information on how to write JSDoc comments, visit the following link.
JSDoc