Learn JavaScript Basics in a Sandbox
In this tutorial, we’ll set up an ultra-simple website to use as a “sandbox” for us to learn the basics of JavaScript. And by the “basics”, I mean the very basic basics. If you’re completely new to JavaScript, this should help you learn some of the things even junior developers take for granted.
Learning Outcome Guide
Here’s a short list of what we will cover in this tutorial.
- Assignment statements
- Variable declarations and initializations
- Constants
- String concatenation
- Math
- Converting from numbers to strings
- Converting from strings to numbers
The Sandbox Page
We’ll use a simple HTML/CSS setup for our sandbox. The whole purpose of this web page is to act as a place where we can see the effect of our code (without relying on logging information to the Developer Tool’s Console).
When our page loads, it will look like this. BTW, I’m basing it on PicoCSS for the base styles and light/dark themes along with Tailwind CSS for custom styles.
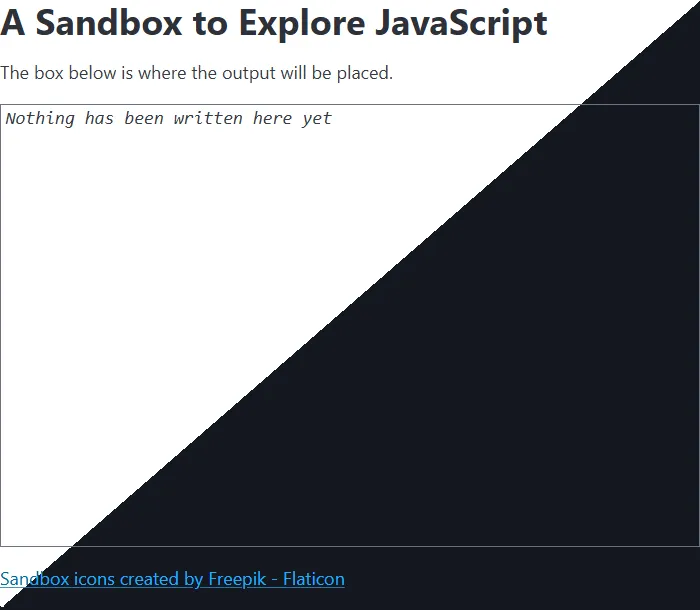
Notice that we have a big “box” area with the text “Nothing has been written here yet”. We’ll be using this as the target for our JavaScript output.
Sandbox Starter Code/Assets
Use the tabs above to get the code and other assets for the Sandbox Starter Kit. Arrange them in this file/folder structure.
Directorycss
- styles.css
Directoryimg
- sandbox-color.png
Directoryjs
- main.js
- index.html
Copy this code and save as index.html
.
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript Sandbox</title> <link rel="shortcut icon" href="./img/sandbox-color.webp" type="image/x-icon"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@picocss/pico@2/css/pico.min.css"> <script src="https://unpkg.com/@tailwindcss/browser@4"></script> <link rel="stylesheet" href="css/styles.css"> <script src="js/main.js" defer></script></head><body class="container h-screen max-h-screen flex flex-col"> <h1>A Sandbox to Explore JavaScript</h1> <p>The box below is where the output will be placed.</p> <output id="sandbox" class="flex-grow w-full overflow-auto block border border-gray-500 custom-inset font-mono px-1 my-2"><em>Nothing has been written here yet</em></output> <footer class="mt-auto"> <a href="https://www.flaticon.com/free-icons/sandbox" title="sandbox icons">Sandbox favicon created by Freepik - Flaticon</a> </footer></body></html>
Copy this code and save as css/styles.css
.
.custom-outset { border-style: inset;}
Copy this code and save as js/main.js
.
console.log('main.js has been loaded');const sandbox = document.querySelector('output#sandbox');
// TODO: Use the space below for playing with JavaScript.
Right-click this image and choose “Save Image As…”, making sure to name it sandbox-color.webp
. We’re using it as a favicon - the icon that appears in the browser’s tab beside the title of the page.

Play and Learn
With our sandbox starter code in place, we can begin experimenting and discovering how JavaScript works. As you walk through this tutorial, remember to save your changes for each step where you write JavaScript code.
To get the backstory on what we will be coding, you should read the following article.
What is a Computer Program? Author TODO: Complete the article + link
Statements in JavaScript
A Statement in JavaScript is a complete instruction. We use a semicolon (;
) at the end to indicate the end of the statement. It works in the same way as a period indicates the end of a sentence (or complete thought) in English.
There are many kinds of instructions we can create in JavaScript. In this part of the tutorial, we’ll write statements that create variables, perform math and change the text on our web page.
-
Launch VS Code and open
main.js
for editing. Then launch the website and view it side-by-side with VS Code. -
Notice in the
main.js
that we have a variable calledsandbox
. This is a reference to the<output>
element from our HTML. Right now, the page shows the hard-coded text inside the<output>
element inindex.html
.Nothing has been written here yet
In the next steps, we will be writing code that uses this element to display content on the web page.
-
Add your first line of code below the
// TODO
comment (don’t remove the existing code). As you save your changes, you should see the text appear on the web page.main.js sandbox.innerText = 'My sandbox is ready to play in.';We’ve just written an assignment statement. Assignment statements use the
=
operator. It works by taking the value on the right-hand side of the operator and storing it in the item on the left-hand side.Your code has now replaced the old value inside
sandbox.innerText
. That’s the purpose of the=
operator. -
Add another line of JavaScript code. This time, we will use a different operator:
+=
main.js sandbox.innerText = 'My sandbox is ready to play in.';sandbox.innerHTML += '<br>A) There are many assignment operators';Notice two things here.
- This time, by using
+=
as the operator, we’ve appended more text to the<output>
element. - We also used
.innerHTML
on the<output>
element so that we can include the<br>
tag to force the remaining text () to appear below our first message. The<br>
tag is the line break.
- This time, by using
-
Let’s do some calculations in JavaScript. We’ll start by calculating the area of a rectangle. Add the following code.
main.js // ... remember to keep the previous codelet leftSide = 3;let topSide = 4;let area;area = leftSide * topSide;- The lines that create and assign values to the
leftSide
andtopSide
variables are called initialilzation statements because they both create the variable and give it a value in the same instruction. - The line that creates the
area
variable is called a declaration statement because it creates the variable without assigning it a starting value. - The last line where we calculate the area is called an assignment statement. We use these to store new information in existing variables.
- The lines that create and assign values to the
-
Right now, our web page does not show the results of our calculation. That’s because we haven’t written the code to display it.
Let’s show our results by adding the following code.
main.js sandbox.innerHTML += '<br>';sandbox.innerHTML += `B) The area of my rectangle is ${area}`;You should see the area’s value of
12
displayed on the page.
We’ve created several lines of code in our sandbox. We’ve explored some simple math and discovered the useful string interpolation syntax.
So far, we’ve only explored a single keyword in the JavaScript language:
let
. We’re going to discover a couple more JavaScript keywords in the next part of this tutorial:const
andtypeof
.All of the other words we’ve written are identifiers. An identifier is simply a name that we or the browser has given for some variable or object.
To learn more about this aspect of JavaScript, check out the following articles.
Data Types, More Math
A lot of times, the information we need to work with is either textual or numeric. JavaScript supports these with the 'string'
and 'number'
data types. There are other built-in data types, but these two will be foundational for almost all of the code you write.
?Add note about the dangers of Primitive Obsession?
Let’s explore how we can manipulate textual and numeric data in our programs.
-
It’s not unusual to see code that has “raw” values in it. In fact, we’ve been using these raw or literal values in earlier parts of this tutorial. A common place to see these are with initialization or assignment statements.
Let’s add a few variables to our code using literal values.
main.js let unitCost = 12.95;let gstRate = 0.05;let currency = '(CAD)'; -
It’s time to do something with these variables. Let’s calculate the price of something. We’ll display the output with a template string
main.js let subTotal = unitCost * 10;// Output the result on another "line"sandbox.innerHTML += '<br>C) ';sandbox.innerHTML += `The subtotal is ${subTotal}. `;sandbox.innerHTML += `The total is ${subTotal + subTotal * gstRate}.`;You should see the following additional text displayed on the page.
C) The subtotal is 129.5. The total is 135.975. -
The math is good for the code we wrote. But the presentation could use some fixing. Make the following changes.
let subTotal = unitCost * 10;let total = subTotal + subTotal * gstRate;// Output the result on another "line"sandbox.innerHTML += '<br>C) ';sandbox.innerHTML += `The subtotal is ${subTotal}. `;sandbox.innerHTML += `The subtotal is $ ${subTotal.toFixed(2)}. `;sandbox.innerHTML += `The total is ${subTotal + subTotal * gstRate}.`;sandbox.innerHTML += `The total is $ ${total.toFixed(2)}.`;We added another variable (
total
) to simplify the placeholder’s expression that calcluated the total amount. In the placeholders, we used the.toFixed()
function that comes will all number types..toFixed()
converts the number to a string with a fixed number of digits precision after the decimal point. Lastly, we also threw in a literal$
in the text to make it clear that we are talking about monetary values.Now the output should look like the following.
C) The subtotal is $ 129.50. The total is $ 135.97.
-
Let’s try some more math, but this time, we’ll use a mixture of strings and numbers.
main.js // Can JavaScript do math with strings and numbers?let numberFive = 5;let stringFive = '5';let answer;answer = numberFive + stringFive;sandbox.innerHTML += '<br>D) ';sandbox.innerHTML += `5 + '5' produces ${answer}`;Did you expect that result? It turns out that JavaScript turned the number into a string and then concatenated the two strings, producing this text.
D) 5 + '5' produces 55
-
Let’s do another calculation. Add the following code.
main.js answer = numberFive * stringFive;sandbox.innerHTML += '<br>E) ';sandbox.innerHTML += `5 * '5' produces ${answer}`;This time, JavaScript turned the string into a number and performed arithmetic. That’s because the multiplcation operator (
*
) is intended for use with numbers, not text.E) 5 * '5' produces 25
-
We can re-try that addition operation. This time let’s use a function that converts strings to numbers:
parseInt()
main.js answer = numberFive + parseInt(stringFive);sandbox.innerHTML += '<br>F) ';sandbox.innerHTML += `5 + parseInt('5') produces ${answer}`;The
parseInt()
will take a string and attempt to convert it to a whole number. There is a similar function if we want to convert to floating-point numbers:parseFloat()
. Here’s the result.F) 5 + parseInt('5') produces 25
-
JavaScript has a keyword that tells us the data type of a variable:
typeof
. Add the following to your growing list of experimental code.main.js sandbox.innerHTML += `<br>G) numberFive is a ${typeof numberFive}`;sandbox.innerHTML += `<br>H) stringFive is a ${typeof stringFive}`;If you are ever curious about the datatype of your variable, just try using the
typeof
operator. -
One last example to try out. We’ve seen the usefulness of literal values (though we should be judicious in their use). Hopefully you’re seeing how variables are even more useful, because we can re-use them to hold newly calculated values (like we did with
answer
).But, what if we wanted a variable that we could re-use in calculations without having its value change? A good example of this is the value for
pi
(3.14150, approximately).main.js const pi = 3.14159; // pi's value is now fixed - it can't changelet radius = 10;sandbox.innerHTML += `<br>I) A circle with radius of ${radius} `;sandbox.innerHTML += `has an area of ${pi * radius * radius} `;sandbox.innerHTML += `and a circumference of ${2 * pi * radius}.`;One of the benefits for using a constant variable (yes, a contradiction in terms) is that it makes our code easier to read and saves us from using lots of hard-coded literals in our scripts.
Conclusion
Assignment statements, variable declarations and initializations, constants, string concatenation, math, converting from numbers to strings and strings to numbers — these are the types of things you should get comfortable writing as a JavaScript programmer.
The concepts we explored in this tutorial form the groundwork on which will will build our JavaScript coding skills. For more information, consult the following article.
Expand for complete code for this tutorial
console.log('main.js has been loaded');const sandbox = document.querySelector('output#sandbox');
// TODO: Use the space below for playing with JavaScript.sandbox.innerText = 'My sandbox is ready to play in.';sandbox.innerHTML += '<br>A) There are many assignment operators';
// ... remember to keep the previous codelet leftSide = 3;let topSide = 4;let area;area = leftSide * topSide;
sandbox.innerHTML += '<br>';sandbox.innerHTML += `B) The area of my rectangle is ${area}`;
let unitCost = 12.95;let gstRate = 0.05;let currency = '(CAD)';let subTotal = unitCost * 10;let total = subTotal + subTotal * gstRate;// Output the result on another "line"sandbox.innerHTML += '<br>C) ';sandbox.innerHTML += `The subtotal is $ ${subTotal.toFixed(2)}. `;sandbox.innerHTML += `The total is $ ${total.toFixed(2)}.`;
// Can JavaScript do math with strings and numbers?let numberFive = 5;let stringFive = '5';let answer;
answer = numberFive + stringFive;sandbox.innerHTML += '<br>D) ';sandbox.innerHTML += `5 + '5' produces ${answer}`;
answer = numberFive * stringFive;sandbox.innerHTML += '<br>E) ';sandbox.innerHTML += `5 * '5' produces ${answer}`;
answer = numberFive + parseInt(stringFive);sandbox.innerHTML += '<br>F) ';sandbox.innerHTML += `5 + parseInt('5') produces ${answer}`;
sandbox.innerHTML += `<br>G) numberFive is a ${typeof numberFive}`;sandbox.innerHTML += `<br>H) stringFive is a ${typeof stringFive}`;
const pi = 3.14159; // pi's value is now fixed - it can't changelet radius = 10;
sandbox.innerHTML += `<br>I) A circle with radius of ${radius} `;sandbox.innerHTML += `has an area of ${pi * radius * radius} `;sandbox.innerHTML += `and a circumference of ${2 * pi * radius}.`;